1.OBTAINING THE TOKEN
- _username: Provided by Spainbox
- _password: Provided by Spainbox
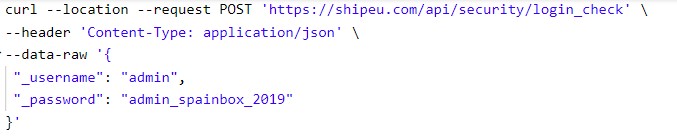
2.USING THE TOKEN
All requests made to the API must be accompanied by the token; for this, the token must be attached in the authorization header of the HTTP request, prefixed by the word Bearer and one space, like this:
Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzUxMiJ……
Notice: The bold characters represent the token
3.RESOURCES
3.1.SENDING ORDERS
The resource to create new orders is: https://shipeu.com/api/v1/order-shipments/new-order
Requests to this resource must have the token in the Authorization header and must be sent with the POST method.
The fields allowed in the request are the following:
Field | Description | Max length | Nullable? |
---|---|---|---|
orderId | Id of the order | 128 | no |
status | Status of the order. Valid values = [completed, cancelled, failed, on-hold, pending, processing, refunded, shipped] | 10 | no |
firstName | First name of the client | 128 | no |
lastName | Last name of the client | 128 | yes |
company | Name of the company if the payer is a company | 128 | yes |
country | The 2-letter code of the country where the order will be sent to. E.g. (ES, FR, DE) | 2 | no |
state | The state where the where the order will be sent to. | 64 | no |
city | The city where the where the order will be sent to. | 64 | no |
addressOne | The main direction of the client. Can be used as street name. | 255 | no |
addressTwo | The secondary direction of the client. Can be used as street number. | 255 | yes |
postCode | Postcode of the client | 25 | no |
billingEmail | The billing email of the client | 255 | yes |
billingPhone | The billing phone of the client | 20 | yes |
shippingMethod | Shipping method of the order | 100 | no |
paymentMethod | Payment method of the order | 100 | no |
orderTotal | Total price of the order. Only numbers allowed | – | yes |
cod | Cost of cash on delivery. Only numbers allowed | – | yes |
lines | Lines (products) of the order. Could be one line or an array of lines. See line specification. | – | no |
shippingCost | Cost of the shipping without taxes. Only numbers allowed | – | yes |
nipNif | NIP/NIF | 128 | yes |
serviceCode | Service code that corresponds to the method of shipment. Service codes available: CHR-EXDO, CTT-EXP, FEDEX-IP, FEDEX-IE | 12 | yes |
Note: When a new order is created, it must have the status“completed”. Shipeu will take the orderswith “completed” status and will change it to “shipped” when the order has been shipped and the tracking info has been set. Later in this manual, will be explained how to obtain those trackings numbers from the orders through the API.
Note 2: The courier for the dispatch of the orders will be set with the one agreed with Spainbox.
ALLOWED VALUES FOR LINES:
Field | Description | Max length | Nullable? |
---|---|---|---|
sku | The sku of the product that is going to be sent in the order | 255 | no |
quantity | The quantity of products that are going to be sent in the order. Only integers allowed. | – | no |
total | Total price | 128 | no |
taxRate | Percent of taxes to apply to the price of the line (only numbers) | – | yes |
linePrice | Product line price (price per product, not the total of the products in the line) | yes |
RESPONSES
Field | Description |
---|---|
status | Status of the response. Possible values: [201, 400, 500] |
error | Error message in case an error has occurred |
data | Returns the previously registered data in case of success (status=200) or returns a list of errors in case an error or errors has occurred (status=400) |
EXAMPLE OF SUCCESS REQUEST
- Json Request for one order and one line:
{
"orderId": "7885-45622-12336",
"firstName": "Jhon",
"secondName": "Doe",
"addressOne": "Av fake",
"addressTwo": "123",
"country": "ES",
"status": "completed",
"state": "Madrid",
"city": "Madrid",
"postCode": "28033",
"shippingMethod": "24h delivery",
"shippingCost": "4.09",
"nipNif": "1234",
"lines": [
{
"sku": "vitanoria",
"quantity": 4,
"total": 20
},
{
"sku": "viswiss",
"quantity": 2,
"total": 25.5
}
]
- Success response:
{
"code": 201,
"data": {
"orderId": "7885-45622-12336",
"firstName": "Jhon",
"secondName": "Doe",
"addressOne": "Av fake",
"addressTwo": "123",
"country": "ES",
"status": "completed",
"state": "Madrid",
"city": "Madrid",
"postCode": "28033",
"shippingMethod": "24h delivery",
"shippingCost": "4.09",
"nipNif": "1234",
"lines": [
{
"sku": "vitanoria",
"quantity": 4,
"total": 20
},
{
"sku": "viswiss",
"quantity": 2,
"total": 25.5
}
]
}
}
- Error response:
{
"code": 400,
"error": "Order validation failed. Please check the errors.",
"data": {
"orderId": [
"This value is already used."
],
"firstName": [
"This value should not be blank."
],
"country": [
"This value should not be blank."
]
}
}
3.2.GETTING TRACKINGS
The resource to get the tracking of the orders in a range of dates is:
https://shipeu.com/api/v1/order-shipments/get-tracking
Requests to this resource must have the token in the Authorization header and must be sent with the GET method. The fields allowed in the request are the following:
Field | Description | Format | Nullable? |
---|---|---|---|
registeredDateStart | Start of the range of dates considering the date of registration of the order | YYYY-MM-DDTHH:MM | no |
registeredDateEnd | End of the range of dates considering the date of registration of the order | YYYY-MM-DDTHH:MM | no |
EXAMPLE OF REQUEST
Get all the orders from July first of 2018 at 00:00 to July second of 2018 at 23:50
https://shipeu.com/api/v1/order-shipments/get-tracking?registeredDateStart=2018-07-01T00:00®isteredDateEnd=2018-07-02T23:50
- Success response:
{
"code": 200,
"data": [
{
"orderId": "12653",
"registeredDate": "2018-07-02T08:21:10+00:00"
},
{
"orderId": "12652",
"tracking": "PQ54A71100012590106230N",
"registeredDate": "2018-07-02T08:21:23+00:00",
"shippedDate": "2018-07-02T09:04:11+00:00"
},
{
"orderId": "12645",
"tracking": "PQ54A71100012600141020T",
"registeredDate": "2018-07-02T08:21:35+00:00",
"shippedDate": "2018-07-02T09:04:11+00:00"
}
]
}
- Error response:
{
"code": 400,
"error": "Start date cannot be greater than end date"
}
3.3.GETTING ORDERS LIST
You can get a list of orders registered in a range of dates and a status. The resource is: https://shipeu.com/api/v1/order-shipments/get-orders
Requests to this resource must have the token in the Authorization header and must be sent with the GET method.
The fields allowed in the request are the following:
Field | Description | Format | Nullable? |
---|---|---|---|
registeredDateStart | Start of the range of dates considering the date of registration of the order | YYYY-MM-DDTHH:MM | no |
registeredDateEnd | Start of the range of dates considering the date of registration of the order | YYYY-MM-DDTHH:MM | no |
status | Status of the order. The values can be: completed -> The order has been registered in Shipeu. shipped -> The order has been shipped | – | no |
EXAMPLE OF REQUEST
Get all the orders shipped from July first of 2018 to July second of 2018
https://shipeu.com/api/v1/order-shipments/get-orders?registeredDateStart=2018-07-01®isteredDateEnd=2018-07-02&status=shipped
- Success response:
{
"code": 200,
"error": null,
"data": [
{
"order_id": "12652",
"status": "shipped",
"first_name": "maria",
"last_name": "manzano dominguez",
"country": "ES",
"state": "BA",
"city": "los santos de maimona",
"address_one": "hernando de soto 12",
"address_two": "",
"post_code": "06230",
"billing_email": "mariamanzanodominguez@gmail.com",
"billing_phone": "655789323",
"shipping_method": "Gratis por Carta ordinaria (10-15 dias)",
"payment_method": "redsys",
"registered_date": "2018-07-02T08:21",
"shipped_date": "2018-07-02T09:04",
"tracking": "PQ0001998457771N",
"lines": [
{
"sku": "1xSKUTEST",
"product_name": "Test product 1",
"quantity": 1,
"total_price": 15.69
},
{
"sku": "1xSKUTEST2",
"product_name": "Test product 2",
"quantity": 5,
"total_price": 23.03
}
]
},
{
"order_id": "12645",
"status": "shipped",
"first_name": "Susana",
"last_name": "Vergara Marquez",
"country": "ES",
"state": "SE",
"city": "Sevilla",
"address_one": "Avenida Del Deporte n 1, Bloque 2,",
"address_two": "4 C",
"post_code": "41020",
"billing_email": "vergaramarquezsusana@gmail.com",
"billing_phone": "664165132",
"shipping_method": "Envío gratuito con entrega 48/72h.",
"payment_method": "redsys",
"registered_date": "2018-07-02T08:21",
"shipped_date": "2018-07-02T09:04",
"tracking": "PQ991884777571T",
"lines": [
{
"sku": "1xSKUTEST3",
"product_name": "Test product 3",
"quantity": 1,
"total_price": 9.99
}
]
}
]
}
- Error response:
{
"code": 400,
"error": "Start date cannot be greater tan end date",
"data": null
}
3.4.GET PRODUCTS STOCK
You can get a list of products with his current stock
The resource is: https://shipeu.com/api/v1/order-shipments/get-stock
Requests to this resource must have the token in the Authorization header and must be sent with the GET method.
https://shipeu.com/api/v1/order-shipments/get-stock
- Success response:
{
"code": 200,
"error": null,
"data": [
{
"sku": "1xSKUTEST",
"name": "Test product",
"stock": 12
},
{
"sku": "1xSKUTEST2",
"name": "Test product 2",
"stock": 76
},
{
"sku": "1xSKUTEST3",
"name": "Test product 3",
"stock": 29
}
]
}
3.5.CANCEL ORDERS
You can cancel an order that has not been sent or already cancelled.
The resource is: https://shipeu.com/api/v1/order-shipments/cancel-order?order-id={orderid}
Requests to this resource must have the token in the Authorization header and must be sent with the PATCH method.
The param “orderid» is the id given to the order.
Note: The order must be in status different from shipped or cancelled in order to be cancelled, otherwise it will return a 400 bad request.
Example request:
https://shipeu.com/api/v1/order-shipments/cancel-order?order-id=test_order_789
- Success response:
{
"code": 200,
"error": null,
"data":
{
"orderId": "test_order_789",
"status": "cancelled"
}
}
- Error response:
{
"code": 400,
"error": "The order was already shipped",
}
3.6.SCHEDULE ORDER PICKUP
You can schedule the pick up of an order.
The resource is:
Sandbox
https://dev.shipeu.com/api/v1/order-shipments/order-return-by-customer
Production
https://shipeu.com/api/v1/order-shipments/order-return-by-customer
Requests to this resource must have the token in the Authorization header and must be sent with the GET method.
The fields allowed in the request are the following:
Field | Description | Format | Length | Nullable? |
---|---|---|---|---|
order-id | Id of the order | – | Max 128 | no |
collected-date | Indicate the date you want generate collection | DD-MM-YYYY | 10 | no |
from-hour | Indicates the time from which wants to generate the collection | 00:00 – 23: 59 | 5 | no |
to-hour | Indicates the last hour at which you want to generate the collection. Must be at least one hour after form-hour | 00:00 – 23: 59 | 5 | no |
RESPONSE
Field | Description |
---|---|
status | Status of the response. Possible values: [200, 400, 500] |
error | Error message in case an error has occurred |
data | Returns the data of the scheduled collection. tracking -> generated tracking code delivery_number -> Associated shipping number pick_up_date -> Pick date from_hour -> from what time is the pickup to_hour -> until what time can you pick up address -> Collection address obtained from the order |
EXAMPLE OF REQUEST
Schedule an order pickup for June 30, 2020, between 8 a.m. to 6 p.m.
https://shipeu.com/api/v1/order-shipments/order-return-by-customer?order-id=FS/ES/2020/4/00988-R&collected-date=30-06-2020&from-hour=08:00&to-hour=18:00
- Success response:
{
"code":200,
"error":"",
"data":{
"tracking":"6300000000239379",
"delivery_number":"69158989",
"pick_up_date":"30062020",
"from_hour":"09:00",
"to_hour":"17:30",
"address":"C\/ Guifr\u00e9 el pel\u00f3s 42","city":"Matar\u00f3"
}
}
- Error response:
{
"code": 400,
"error": "The end time must be at least one hour higher than the start time."
}
3.7.SCHEDULE CTT ORDER PICKUP
The resource is:
Requests to this resource must have the token in the Authorization header and must be sent with the GET method.
The fields allowed in the request are the following:
Field
|
Description
|
Format
|
Length
|
Nullable?
|
---|---|---|---|---|
order-id
|
Id of the order
|
–
|
Max 128
|
no
|
collected-date
|
Indicate the date you want
generate collection |
DD-MM-YYYY
|
10
|
no
|
from-hour
|
Indicates the time from which
wants to generate the collection |
00:00 – 23: 59
|
5
|
no
|
to-hour
|
Indicates the last hour at which
you want to generate the collection. Must be at least one hour after form-hour |
00:00 – 23: 59
|
5
|
no
|
RESPONSE
Field
|
Description
|
---|---|
status
|
Status of the response.
Possible values: [200, 400, 500] |
error
|
Error message in case an error has occurred
|
data
|
Returns the data of the scheduled collection.
tracking -> generated tracking code delivery_number -> Associated shipping number pick_up_date -> Pick date from_hour -> from what time is the pickup to_hour -> until what time can you pick up address -> Collection address obtained from the order |